Alli Cognitive Search User Guide - Javascript SDK integration
Alli Cognitive Search SDK integration is simple. Just copy and paste the following code to your website and update necessary information such as API Key or styles.
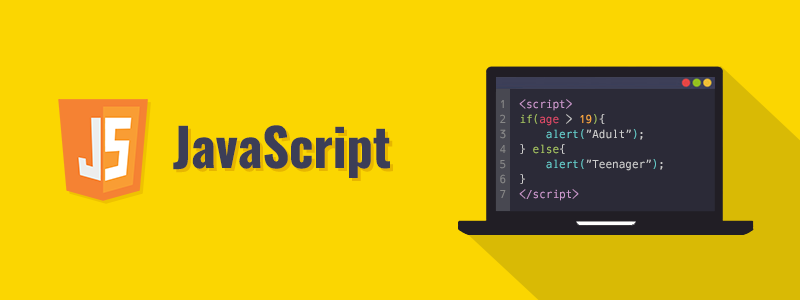
Alli Cognitive Search SDK integration is simple. Just copy and paste the following code to your website and update necessary information such as API Key or styles.
Please note that our Cognitive Search SDK is a very early version, so the content will be continuously updated.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, minimum-scale=1, initial-scale=1.0, shrink-to-fit=no"
/>
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<!-- Alli search SDK stylesheets -->
<link href="https://sdk.alli.ai/0.0/alli.search.css" rel="stylesheet">
<link href="https://sdk.alli.ai/0.0/alli.search.__carousel.css" rel="stylesheet">
<!-- Alli search SDK polyfill for IE 11 -->
<script src="https://polyfill.io/v3/polyfill.min.js?features=Object.assign%2Cfetch%2CArray.from%2CString.prototype.startsWith%2CrequestAnimationFrame%2CArray.prototype.find%2CArray.prototype.findIndex%2CString.prototype.endsWith%2CArray.prototype.fill"></script>
<!-- Alli search SDK javascript file -->
<script src="https://sdk.alli.ai/0.0/alli.search.js"></script>
</head>
<body>
<div id="app"></div>
<script>
var apiKey = 'YOUR_API_KEY';
var app = document.getElementById('app'); // Use your own element
function isMobileOS() {
return /Android|webOS|iPhone|iPad|iPod|Opera Mini/i.test(navigator.userAgent);
}
var isMobile = isMobileOS();
var auth = new AlliSearch.AlliAuth('YOUR_API_KEY');
auth.userid ='YOUR_USER_ID';
var client = new AlliSearch.AlliSearchClient({
apiKey: 'YOUR_API_KEY',
debug: true,
auth: auth,
});
var search = new AlliSearch(client);
client.project().then(function (result) {
if (result.data && result.data.project) {
var faq = result.data.project.searchSdkSettings.faq;
var mrc = result.data.project.searchSdkSettings.mrc;
search.input = Object.assign({}, search.input, {
settings: Object.assign({}, search.input.settings, {
faq: Object.assign({}, search.input.settings && search.input.settings.faq, {
faqSimilarQuestionCount: faq.count,
}),
mrc: Object.assign({}, search.input.settings && search.input.settings.mrc, {
displayCount: mrc.count,
}),
}),
});
AlliSearch.UI.__unstableRender__(search, app, {
SearchForm: {
input: { placeholder: 'Type your question' },
submit: { children: 'Search' },
},
QnA: {
// Q&A section show/hide - if true, Q&A section will not show
disabled: false,
// Q&A section title - opts includes count and query
title: function (opts) {
return 'Q&A';
},
// Text to display when Q&A search results are loading
loading: 'Loading...',
// Text to display when there are no Q&A search results
empty: 'No results found',
// Text to display when Q&A search results have failed to load
error: function (errs) {
return 'Something went wrong!';
},
result: {
clamp: faq.maxHeight || undefined,
// Q&A search result preview button text
preview: 'Preview',
// Q&A search result read more/show less button text
truncation: function (opts) {
return opts.truncated ? 'Read More' : 'Show Less';
},
},
__variant:
faq.carouselOnMobile && isMobile ? 'carousel' : 'default',
},
Documents: {
// Documents section show/hide - if true, documents section will not show
disabled: false,
// Documents section title - opts includes count and query
title: function (opts) {
return 'Documents';
},
// Text to display when document search results are loading
loading: 'Loading...',
// Text to display when there are no document search results
empty: 'No results found',
// Text to display when document search results have failed to load
error: function (errs) {
return 'Something went wrong!';
},
result: {
clamp: mrc.maxHeight || undefined,
// Document search result preview button text
preview: 'Preview',
// Document search result read more/show less button text
truncation: function (opts) {
return opts.truncated ? 'Read More' : 'Show Less';
},
},
__variant:
mrc.carouselOnMobile && isMobile ? 'carousel' : 'default',
},
DocumentPreview: {
// Whether to show document preview full screen
fullScreen: isMobile,
// styles applied to highlighted texts in document preview
highlight: {
backgroundColor: 'rgba(0,0,0,0.12)',
},
loading: 'Loading...',
empty: 'No preview available',
error: function (errs) {
return 'Something went wrong!';
},
},
RichText: {
customStyleMap: {
// styles applied to highlighted texts in answers
HIGHLIGHT: {
backgroundColor: 'rgba(0,0,0,0.12)',
},
},
},
});
}
});
</script>
</body>
</html>
Please replace 'YOUR_API_KEY' to your Alli project's API key that you can find under Settings > General menu. Make sure to use the SDK key, not the REST API key.
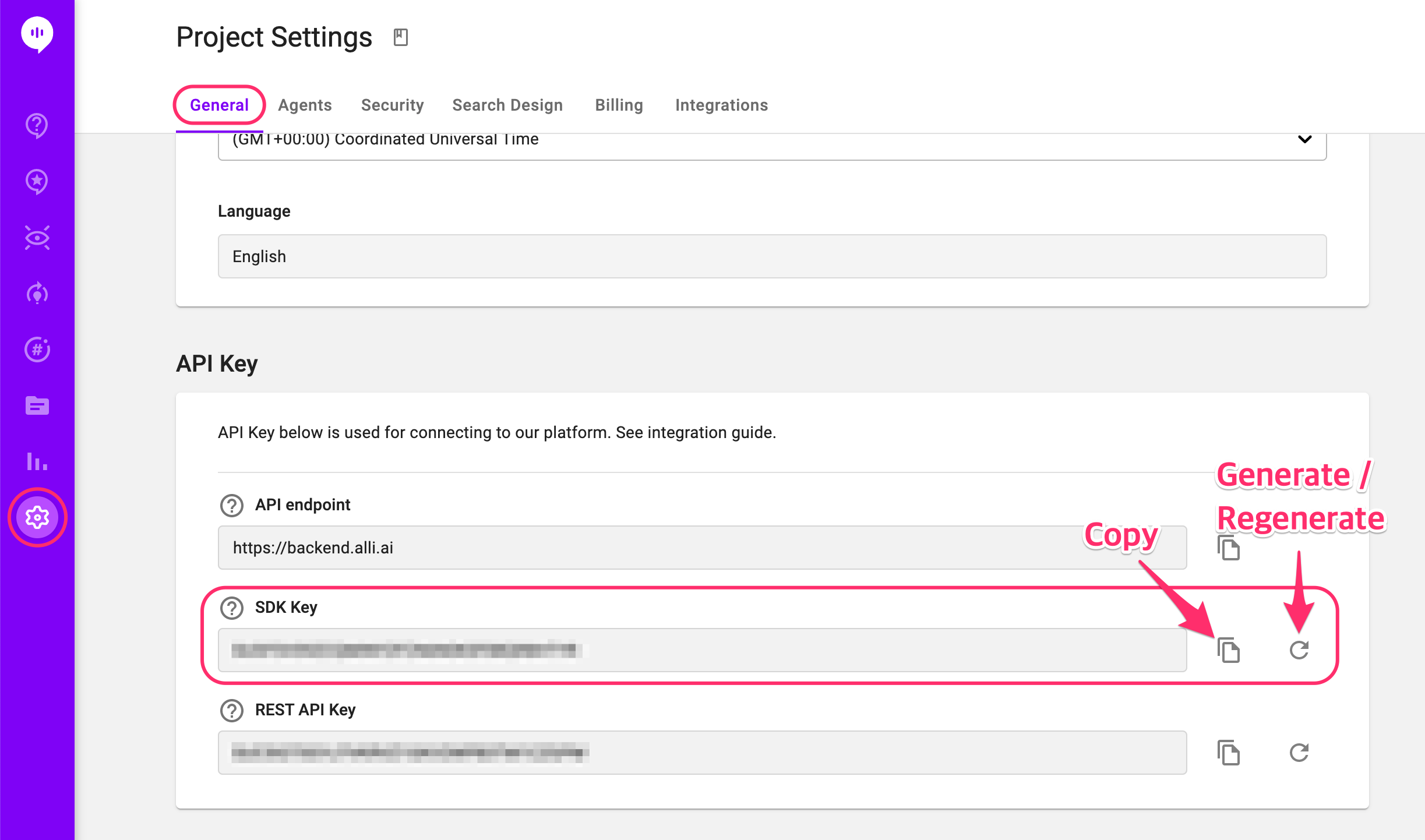
Below are more details about each elements in the SDK.
Head
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, minimum-scale=1, initial-scale=1.0, shrink-to-fit=no"
/>
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<!-- Alli search SDK stylesheets -->
<link href="https://sdk.alli.ai/0.0/alli.search.css" rel="stylesheet">
<link href="https://sdk.alli.ai/0.0/alli.search.__carousel.css" rel="stylesheet">
<!-- Alli search SDK polyfill for IE 11 -->
<script src="https://polyfill.io/v3/polyfill.min.js?features=Object.assign%2Cfetch%2CArray.from%2CString.prototype.startsWith%2CrequestAnimationFrame%2CArray.prototype.find%2CArray.prototype.findIndex%2CString.prototype.endsWith%2CArray.prototype.fill"></script>
<!-- Alli search SDK javascript file -->
<script src="https://sdk.alli.ai/0.0/alli.search.js"></script>
</head>
- You can resize overall UI.
- For the stylesheets, you can use your own CSS if you want.
- The polyfill part is for IE 11 compatibility. Please customize accordingly considering your environment.
- Current JS SDK version is 0.0 as this version of SDK is a prototype.
Loading SDK
<body>
<div id="app"></div>
<script>
var apiKey = 'YOUR_API_KEY';
var app = document.getElementById('app'); // Use your own element
function isMobileOS() {
return /Android|webOS|iPhone|iPad|iPod|Opera Mini/i.test(navigator.userAgent);
}
var isMobile = isMobileOS();
var auth = new AlliSearch.AlliAuth('YOUR_API_KEY');
auth.userid ='YOUR_USER_ID';
var client = new AlliSearch.AlliSearchClient({
apiKey: 'YOUR_API_KEY',
debug: true,
auth: auth,
});
var search = new AlliSearch(client);
client.project().then(function (result) {
if (result.data && result.data.project) {
var faq = result.data.project.searchSdkSettings.faq;
var mrc = result.data.project.searchSdkSettings.mrc;
search.input = Object.assign({}, search.input, {
settings: Object.assign({}, search.input.settings, {
faq: Object.assign({}, search.input.settings && search.input.settings.faq, {
faqSimilarQuestionCount: faq.count,
}),
mrc: Object.assign({}, search.input.settings && search.input.settings.mrc, {
displayCount: mrc.count,
}),
}),
});
</script></body>
- The SDK identifies the project with the API key, and import Search Design settings from the dashboard. Please see the end of this user guide to learn more about the Search Design settings.
- For app, you can use your own element.
- You can use user ID to specify the user. Simply replace YOUR_USER_ID for auth.userid to the ID you want to use.
- Function 'isMobileOS' is used to identify mobile environment so the SDK can support carousel UI for mobile users.
- You can also override some settings such as faq.count or mrc.count. Each setting decides how many search results are suggested for the Q&A search and the Documents search.
AlliSearch.UI.__unstableRender__
SearchForm
<script>
SearchForm: {
input: { placeholder: 'Type your question' },
submit: { children: 'Search' },
}
</script>
- You can customize the placeholder text for the search input field and the text on the search button.
QnA
<script>
QnA: {
// Q&A section show/hide - if true, Q&A section will not show
disabled: false,
// Q&A section title - opts includes count and query
title: function (opts) {
return 'Q&A';
},
// Text to display when Q&A search results are loading
loading: 'Loading...',
// Text to display when there are no Q&A search results
empty: 'No results found',
// Text to display when Q&A search results have failed to load
error: function (errs) {
return 'Something went wrong!';
},
result: {
clamp: faq.maxHeight || undefined,
// Q&A search result preview button text
preview: 'Preview',
// Q&A search result read more/show less button text
truncation: function (opts) {
return opts.truncated ? 'Read More' : 'Show Less';
},
},
__variant:
faq.carouselOnMobile && isMobile ? 'carousel' : 'default',
}
</script>
- If disabled is true, the SDK won't show the Q&A search results.
- You can customize the title of the Q&A search results section in the function(opts). opts.count is the number of search results, and opts.query is the search query.
- You can use function(errs) to customize error messages. Errors can be SDKError, GraphQLError, javascript error, apollo error types or unidentified.
- You can customize the text strings in the UI for the title of the search results, loading, no results found, error messages, result preview button, expand button, and collapse button.
- The title of the Q&A search results section can be customized in the function(opts). opts.count is the number of search results, and opts.query is the search query.
- The error messages can be customized using function(errs). Errors can be SDKError, GraphQLError, javascript error, apollo error types or unidentified. - clamp is for the max height of each search result before it's expanded.
- __variant is to support carousel UI for mobile users. Current example is to follow the Search Design settings in the dashboard. Please see the end of this user guide to learn more about the Search Design settings.
Documents
The elements here are pretty much the same with the ones under the QnA above.
<script>
Documents: {
// Documents section show/hide - if true, documents section will not show
disabled: false,
// Documents section title - opts includes count and query
title: function (opts) {
return 'Documents';
},
// Text to display when document search results are loading
loading: 'Loading...',
// Text to display when there are no document search results
empty: 'No results found',
// Text to display when document search results have failed to load
error: function (errs) {
return 'Something went wrong!';
},
result: {
clamp: mrc.maxHeight || undefined,
// Document search result preview button text
preview: 'Preview',
// Document search result read more/show less button text
truncation: function (opts) {
return opts.truncated ? 'Read More' : 'Show Less';
},
},
__variant:
mrc.carouselOnMobile && isMobile ? 'carousel' : 'default',
}
</script>
- If disabled is true, the SDK won't show the Q&A search results.
- You can customize the title of the Documents search results section in the function(opts). opts.count is the number of search results, and opts.query is the search query.
- You can use function(errs) to customize error messages. Errors can be SDKError, GraphQLError, javascript error, apollo error types or unidentified.
- You can customize the text strings in the UI for the title of the search results, loading, no results found, error messages, result preview button, expand button, and collapse button.
- The title of the Documents search results section can be customized in the function(opts). opts.count is the number of search results, and opts.query is the search query.
- The error messages can be customized using function(errs). Errors can be SDKError, GraphQLError, javascript error, apollo error types or unidentified. - clamp is for the max height of each search result before it's expanded.
- __variant is to support carousel UI for mobile users. Current example is to follow the Search Design settings in the dashboard.
DocumentPreview
<script>
DocumentPreview: {
// Whether to show document preview full screen
fullScreen: isMobile,
// styles applied to highlighted texts in document preview
highlight: {
backgroundColor: 'rgba(0,0,0,0.12)',
},
loading: 'Loading...',
empty: 'No preview available',
error: function (errs) {
return 'Something went wrong!';
},
}
</script>
- DocumentPreview decides the style of the preview modal appears when you click the preview button in a Documents search result.
- The estimated answer part is highlighted in the preview, and you can customize the style of the highlighted text. You can use your own CSS or use style objects of your choice.
- You can customize the text strings in the UI for loading, no preview available, and error messages.
- The error messages can be customized using function(errs). Errors can be GraphQLError type or unidentified.
RichText
<script>
RichText: {
customStyleMap: {
// styles applied to highlighted texts in answers
HIGHLIGHT: {
backgroundColor: 'rgba(0,0,0,0.12)',
},
},
}
</script>
- RichText decides the style of the highlighted text (the correct answer part) in the search result. You can use your own CSS or use style objects of your choice.
Search Design Settings
Search Design settings can be found under Settings > Search Design.
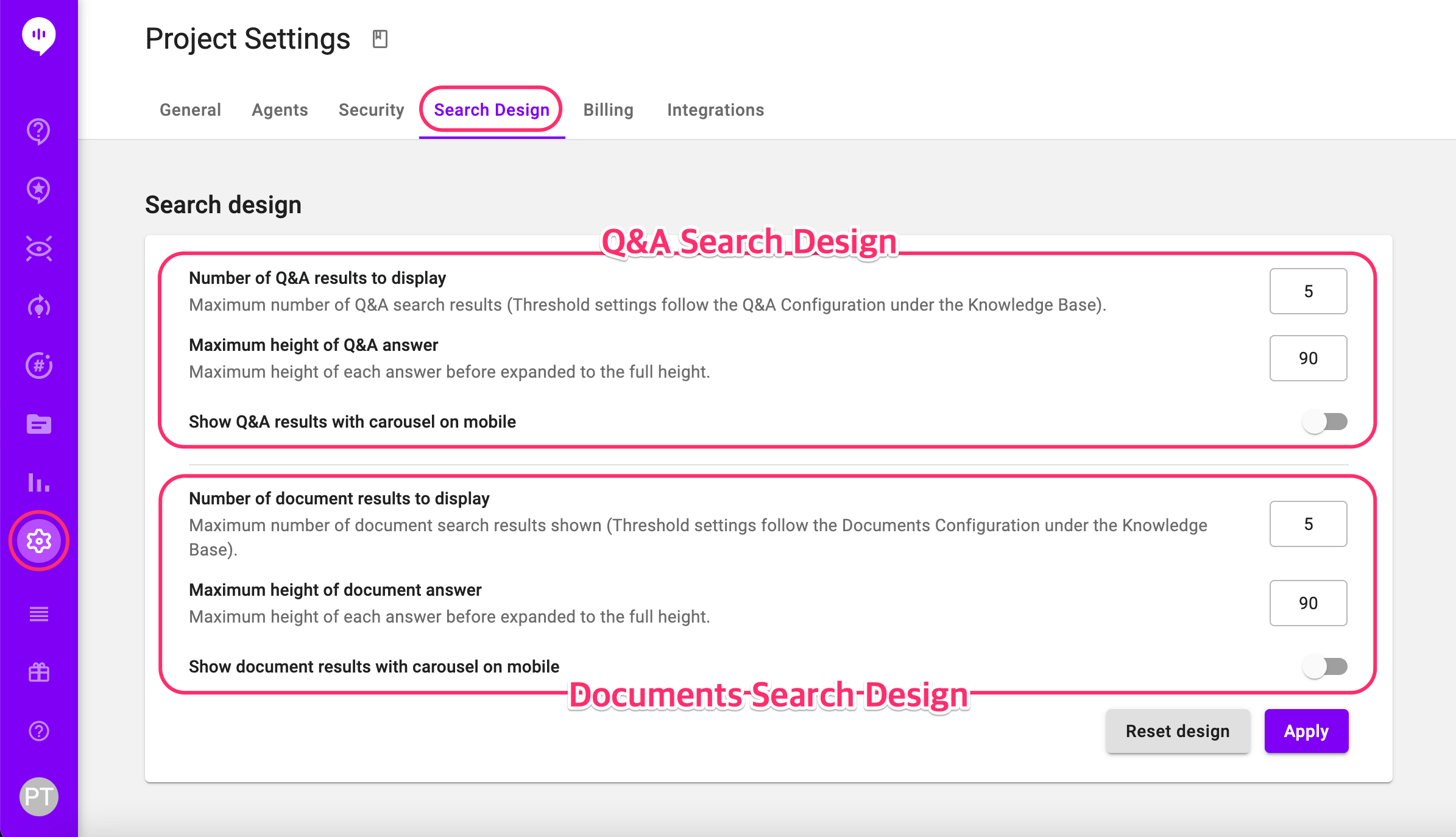
There are two sections under the Search Design settings. Upper section changes the settings for the Q&A search, and the lower section the Documents search. You can change number of answers and maximum height of an answer, and if you turn on the toggle for the 'Show results with carousel on mobile' option, the search results will be displayed in carousel UI and can be navigated by swiping left or right.
CSS Editing
Other design elements can be edited via editing CSS. Below guide introduces how to customize the search box border color, the call to action button color, and the SDK title&logo.
Search box border customization

Step 1 . Use the ".alli-search_SearchForm__input" in <style> tag section

Step 2. Change the border color by replacing the color code under "border" style code by putting the Hex Color Code (eg: #FFFFFF) or Decimal Code (eg: rgb(255,255,255) you desire.
Call to action text color customization

Step 1. Use ".alli-search_Button__label" in <style> tag section
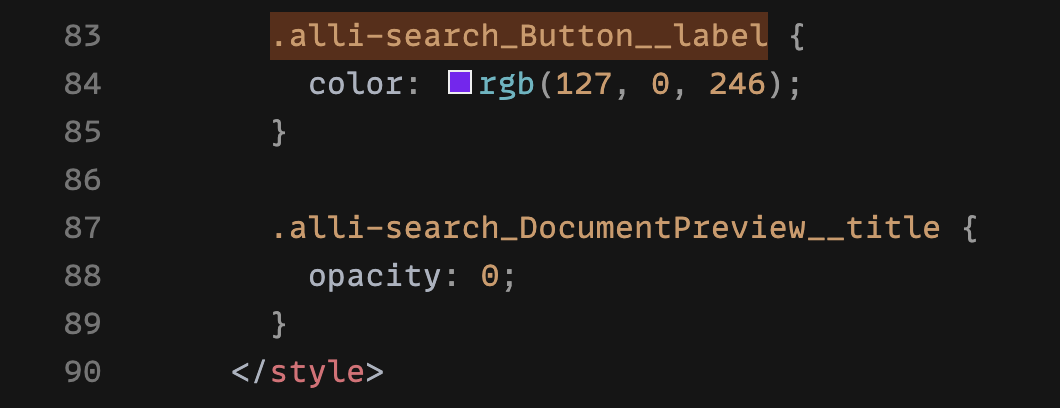
Step 2. Change the border color by replacing the color code under "border" style code by putting the Hex Color Code (eg: #FFFFFF) or Decimal Code (eg: rgb(255,255,255) you desire.